Table of Contents
Introduction to functions
How to define and call functions in C++? Function and their types in C++ are very important. Functions in C++ make program more compact. In programming, if we want to do some tasks repeatedly we prefer using loops but its really difficult to write down the same loops whenever we want to perform that specific task. So, rather than writing those long statements its better to go for some approach where we can bound that repeated task within some brackets and then “name” them as “function”. So whenever we want to perform that task anywhere in the program. we can call that function.
Flow of a function
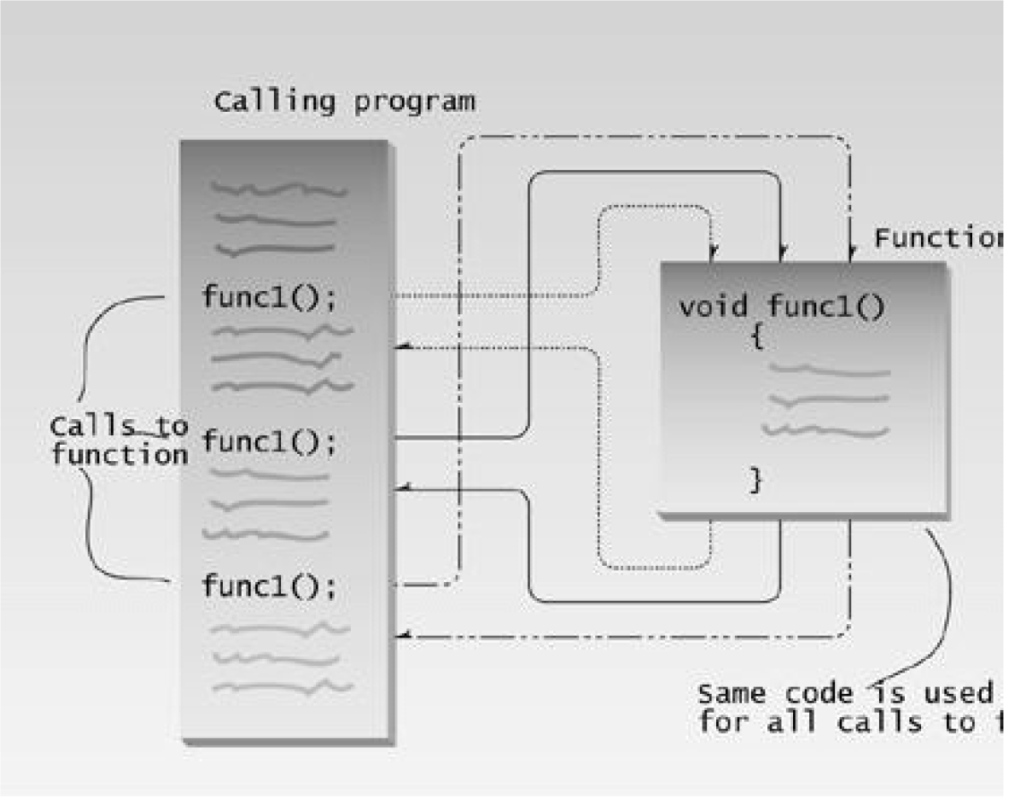
Three things that are linked with the functions are:
- Function prototype (optional)
- Function definition.
- Function Call.
The function prototype is also called function declaration. How many functions you want to include in your program, you need to declare them first. The prototype is an optional thing if you want to define the function before the main program. If the function definition is included after the main function then its necessary to declare the function before main program.
Prototype of functions
The syntax for function prototype is as follows:
return type function_name (types of variables);
e.g.,
void sum(int, int);
so this type of function does not return any value and takes two arguments of integer type.
Function Definition
what task you expect to perform by the function is defined in the function definition. its syntax is:
return type functionname(list of variables with their data type)
{
set of statements
}
example:
int sum (int a, int b)
{
return a+b;
}
Function Call
Function call is made when we need its value. We can make multiple calls to a function by passing arguments to it. the syntax for function call is:
function_name( arguments value);
sum(x,y);
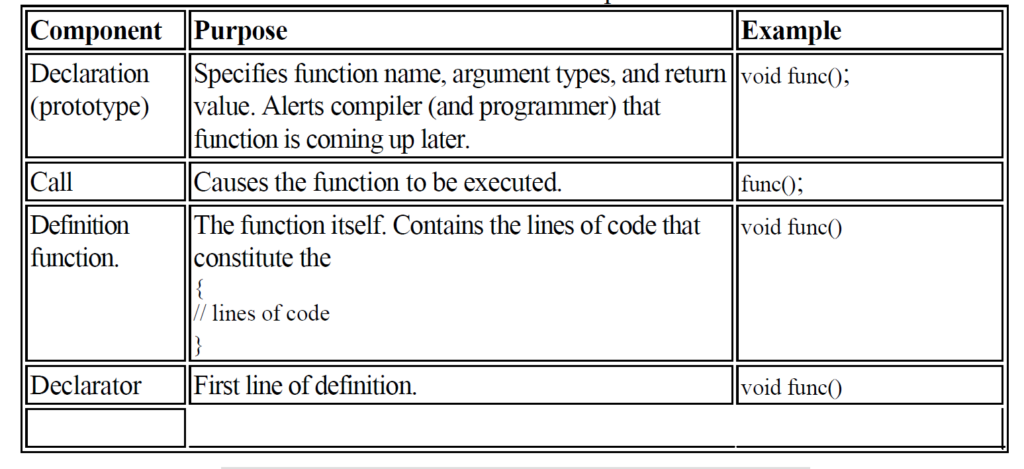
Passing arguments to a function
There are two ways to pass arguments to a function.
- pass by value
- pass by reference
Pass by value:
when we pass the argument by value then logically its copy is passed to the definition of the function and the original value of the argument that is passed is not updated/changed.
Pass by reference:
The syntax to pass the arguments by reference is (int &count) which means count is referred as integer. Whenever we pass the arguments by reference then the actual value of the arguments is passed to the function and whatever the changes are introduced through the definition of the function to that argument are copied to the original variable. The following example illustrates this concept.
Example
#include<iostream.h>
#include<conio.h>
void f(int & n)
{
cout<<++n<<endl;
}
int main()
{
clrscr();
int x = 2;
f(x);
cout << x;
getch();
return 0;
}
initially the value of x=2 but when we call the function then as x has been passed as referenced argument its value will be incremented and it will become equal to 3.
Function Overloading
You program may contain multiple functions with the same name but taking arguments of different data types. This is called function overloading. They usually perform the same task on different data types. The following example illustrates this concept.
Example
#include<iostream.h>
#include<conio.h>
int square(int a)
{
return a*a;
}
double square( double b)
{
return b*b;
}
void main
{
crlscr();
int a;
double b;
cin>>a;
cout<<square(a);
cin>>b;
cout<<square(b);
getch();
}
Compiler differentiates between these two function from their return type and the number of arguments they take.
Functions and their types in c++
Functions and their types in C++ are discussed here in detail. Usually in classes friends functions are used.
Friend Functions
Encapsulation property in object oriented property demands privacy. It means the non member functions are not allowed to access the private or protected data of an object of a class. But this rigid behavior needs little flexibility just to avoid inconvenience.
Friends as bridges
Friend functions provide bridging. If there is some function that wants to operate on the objects of different classes then there should be some possible way through which it can access the private data. Here the objects of two different classes might be passed as arguments to that function. For understanding this, consider the following example.
class gema;
class mega
{
private:
int dataa;
public:
mega():dataa(5)
{
}
friend int friendfunction(mega, gema);
};
class gema
{
private:
int dataa;
public:
gema():dataa(2)
{
}
friend int friendfunction(mega,gema);
};
int friendfunction(mega a,gema b)
{
return (a.dataa+b.dataa);
}
void main()
{
clrscr();
mega bb;
gema cc;
cout<<friendfunction(bb,cc)<<endl;
getch();
}
Here two classes are used gema and mega (you can name them any). Constructor is used for initializing the data member of both classes with constant values. The friendfunction() is made to have access of dataa item of both classes with the reserved keyword ‘friend’. It can be seen that the object of both classes has been passed as argument to friendfunction() for accessing the private data items. Here the task of friend unction is to add the values of both data items provided to it. The call of function is made inside the main where it only adds two values.
Example of functions and their types in C++
- Write a function double quadratic (double x) that computes function f(x) with x as the only input parameter and returns f(x) = ax2 + bx + c with a = 1.0, b = 2.0, and c = 1.0
Solution:
#include<iostream.h>
#include<conio.h>
#include<math.h>
double quadratic (double x)
{
int a=1, b=2,c=1;
return a*pow(x,2)+b*x+c;
}
void main()
{
clrscr();
double y;
cin>>y;
cout<<quadratic(y);
getch();
}
2. Write a function distance2 that calculates the distance between two points (x1, y1) and (x2, y2) in the plane. Put all numbers and return types in double. Use (3.0, 0.0) and (0.0, 4.0) as one pair of input to verify your program.
solution:
double distance2(int x1,int y1,int x2,int y2)
{
return sqrt(pow(x2-x1,2)+pow(y2-y1,2));
}
void main()
{
cout<<distance2(3,0,0,4);
}
3. Use the rand function to calculate the frequency of each face of a dice when it is rolled 500 times.
Solution:
#include<stdlib.h>
#include<time.h>
void randomgen(void)
{
int number,f1=0,f2=0,f3=0,f4=0,f5=0,f6=0;
srand(time(0));
for(int i=0;i<500;i++)
{
number=rand()%6;
if(number==1)
f1++;
else
if(number==2)
f2++;
else
if(number==3)
f3++;
else
if(number==4)
f4++;
else
if(number==5)
f5++;
else
f6++;
}
cout<<“face 1 occurred”<<f1<<“times”<<endl;
cout<<“face 2 occurred”<<f2<<“times”<<endl;
cout<<“face 3 occurred”<<f3<<“times”<<endl;
cout<<“face 4 occurred”<<f4<<“times”<<endl;
cout<<“face 5 occurred”<<f5<<“times”<<endl;
cout<<“face 6 occurred”<<f6<<“times”<<endl;
}
void main()
{
clrscr();
randomgen();
getch();
}
4. Write down a function that comoutes either the guven number is percect or not.
Solution:
int testit(int n)
{
int sum=0;
for(int i=1;i<n;i++)
{
if(n%i==0)
sum=sum+i;
}
return sum;
}
void main()
{
int x;
clrscr();
cout<<“enter the number for testing it is perfect or not”<<endline;
cin>>x;
if(x==testit(x))
cout<<“it is a perfect number “;
else
cout<<“not a perfect number”;
getch();
}
for more detail follow the given links:
Define function overloading in c++
How to pass arguments to a function by reference?
Also read here scope of a variable
http://Scope of a variable in programming with c++
3 thoughts on “How to define and call functions in C++?”