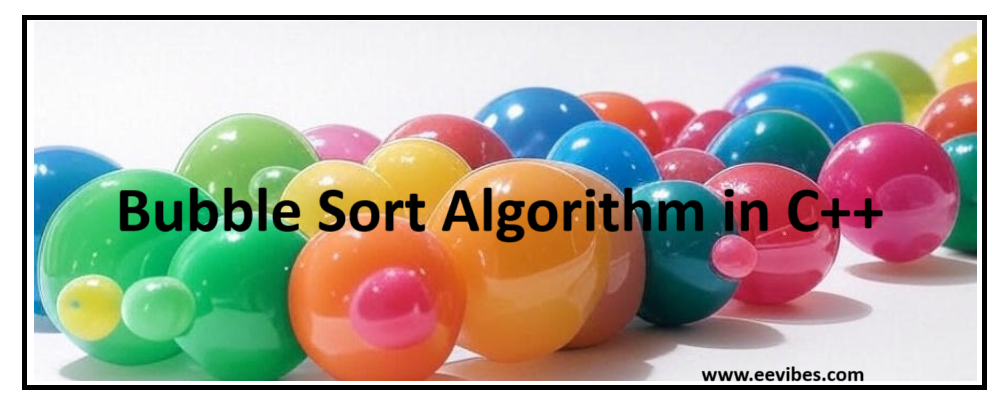
Bubble Sort
Bubble sort in programming is used for arranging the array elements either in ascending or descending orders. This is the simplest algorithm in programming and easily implementable.
Working of Bubble Sort Algorithm
In this algorithm, we start making the comparison of first two elements of array. If the first element is greater than the second element, we will swap them in case of arranging the elements in ascending order. Hence we keep comparing and swapping the consecutive elements until the first round is completed.
After the completion of first round, the largest element is present at the end of the array. So, now we will move the second largest element of an array in the second round by using the same approach of comparison and swapping.
How many Passes are required in Bubble Sort?
Now, the question arises, that how many passes or the rounds are required? The maximum number of passes or the rounds required for performing the bubble sort algorithm is n-1 where n is the size of array. Suppose that we want to arrange the array of 10 elements, so the maximum number of passes are n-1=9. Although, there is the probability that the array gets sorted in less than n-1 passes.
Bubble Sort Example
C++ Program for Bubble Sort Algorithm
Video Lecture for Bubble Sort Algorithm
Here is the complete description of how bubble sort algorithm works. I have taken an example of 10 array elements, where the array size is declared using const int size=10 command. Then a nested for loop is used for performing sorting using bubble sort. The outer loop is used for total rounds/passes which is n-1 in this case. While the inner loop is used for making continuous comparison and swapping where required.