Introduction to the project
How to design a clock project in c++ ? write a program that will take quiz related to any topic in given time.
- Show a clock with the help of graphics library
- Show the quiz questions related to any topic like C++ language.
- Take user’s input as the answer of the question.
- Check whether the answer is correct or not.
- Matching the answer and print result.
- Clock stops and quiz ends automatically after certain time.
C++ code for clock designing project
Code
#include<graphics.h>
#include<cmath>
#include<ctime>
#include <iostream>
#define PI 3.1415
using namespace std;
int main(){
int pressed=0;
tm *ltm ;
int clock;
int result;
clock=initwindow(500,800,”Clock”);
setbkcolor(15);
setcolor(0);
settextstyle(3, 0, 1);
cleardevice();
int page=0;
int ss=0;
int ans;
int loo=1;
int los=0;
while(loo==1){
setactivepage(page);
setvisualpage(1-page);
if(pressed==0){
cleardevice();
}
circle(250,250,200);
circle(250,250,5);
outtextxy(250+180*sin(PI/6)-5, 250-180*cos(PI/6)-5, “1”);
outtextxy(250+180*sin(2*PI/6)-5, 250-180*cos(2*PI/6)-5, “2”);
outtextxy(250+180*sin(3*PI/6)-5, 250-180*cos(3*PI/6)-5, “3”);
outtextxy(250+180*sin(4*PI/6)-5, 250-180*cos(4*PI/6)-5, “4”);
outtextxy(250+180*sin(5*PI/6)-5, 250-180*cos(5*PI/6)-5, “5”);
outtextxy(250+180*sin(6*PI/6)-5, 250-180*cos(6*PI/6)-5, “6”);
outtextxy(250+180*sin(7*PI/6)-5, 250-180*cos(7*PI/6)-5, “7”);
outtextxy(250+180*sin(8*PI/6)-5, 250-180*cos(8*PI/6)-5, “8”);
outtextxy(250+180*sin(9*PI/6)-5, 250-180*cos(9*PI/6)-5, “9”);
outtextxy(250+180*sin(10*PI/6)-5, 250-180*cos(10*PI/6)-5, “10”);
outtextxy(250+180*sin(11*PI/6)-5, 250-180*cos(11*PI/6)-5, “11”);
outtextxy(250+180*sin(12*PI/6)-5, 250-180*cos(12*PI/6)-5, “12”);
if(pressed==0){
time_t now = time(0);
ltm = localtime(&now);
system(“cls”);
}
// Hour Hand
setcolor(RED);
line(250,250,250+150*sin(ltm->tm_hour * PI/6),250-150*cos(ltm->tm_hour * PI/6));
// Minute Hand
setcolor(GREEN);
line(250,250,250+190*sin(ltm->tm_min * PI/30),250-190*cos(ltm->tm_min * PI/30));
// Secod Hand
setcolor(BLUE);
line(250,250,250+150*sin(ltm->tm_sec * PI/30),250-150*cos(ltm->tm_sec * PI/30));
outtextxy(10,500,”Who is the creator of the C++ language?”);
outtextxy(10,560,”A. Dennis Ritchie”);
outtextxy(10,590,”B. Ken Thompson”);
outtextxy(10,620,”C. Bjarne Stroustrup”);
outtextxy(10,650,”D. Brian Kernighan”);
if(GetAsyncKeyState(0x41)){
pressed=1;
cleardevice();
setbkcolor(3);
outtextxy(10,680,”Wrong Answer”);
}
if(GetAsyncKeyState(0x42)){
pressed=1;
cleardevice();
setbkcolor(3);
outtextxy(10,680,”Wrong Answer”);
}
if(GetAsyncKeyState(0x43)){
pressed=1;
cleardevice();
setbkcolor(3);
outtextxy(10,680,”Answer is correct”);
}
if(GetAsyncKeyState(0x44)){
pressed=1;
cleardevice();
setbkcolor(3);
outtextxy(10,680,”Answer is false”);
}
if(pressed==0){
ss=ss+1;
if(ss>350){
pressed=1;
outtextxy(10,680,”Time up”);
}
}
delay(10);
page = 1-page;
}
getch();
closegraph();
return 0;
}
Flow chart for project
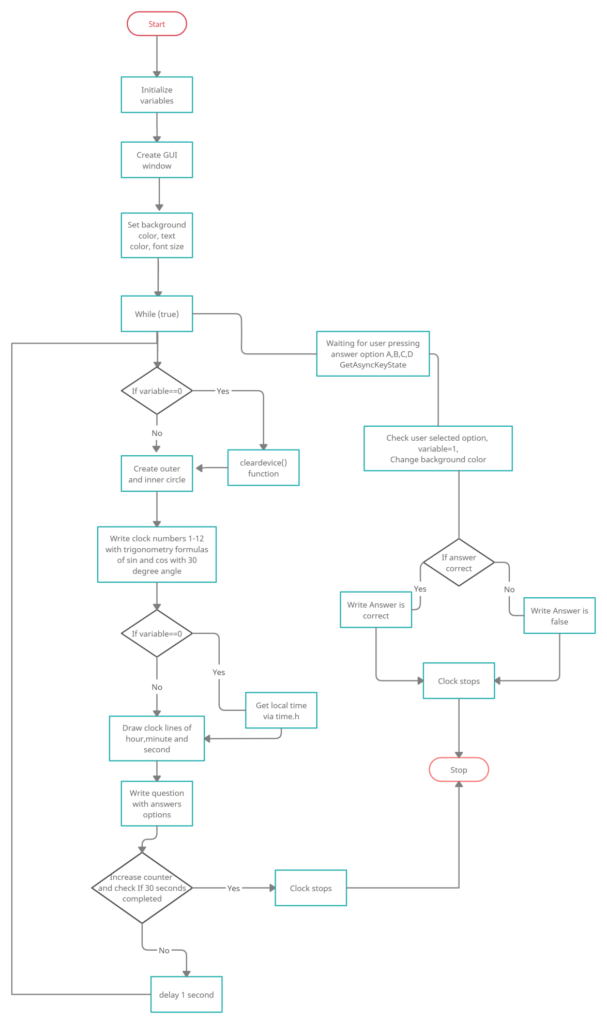
Output of the project
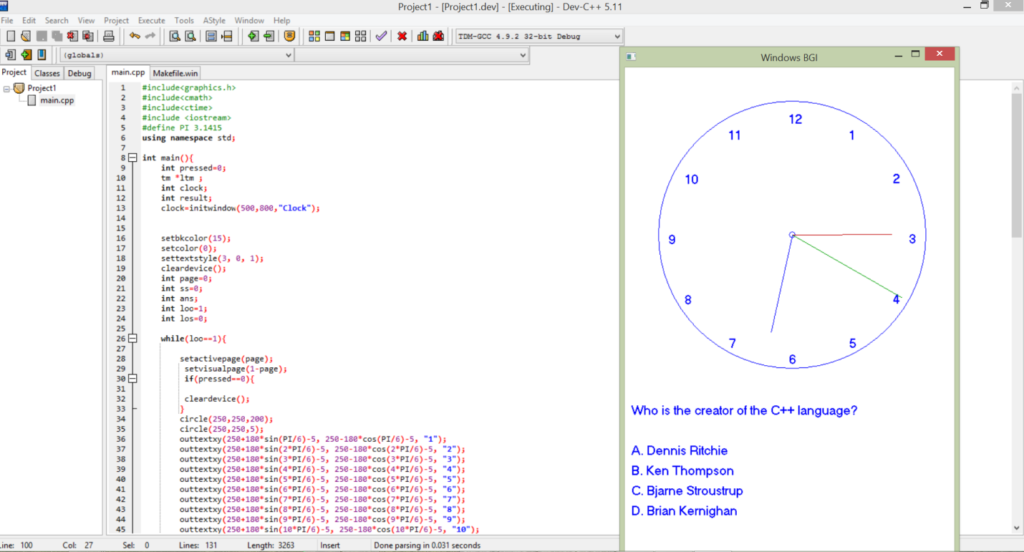
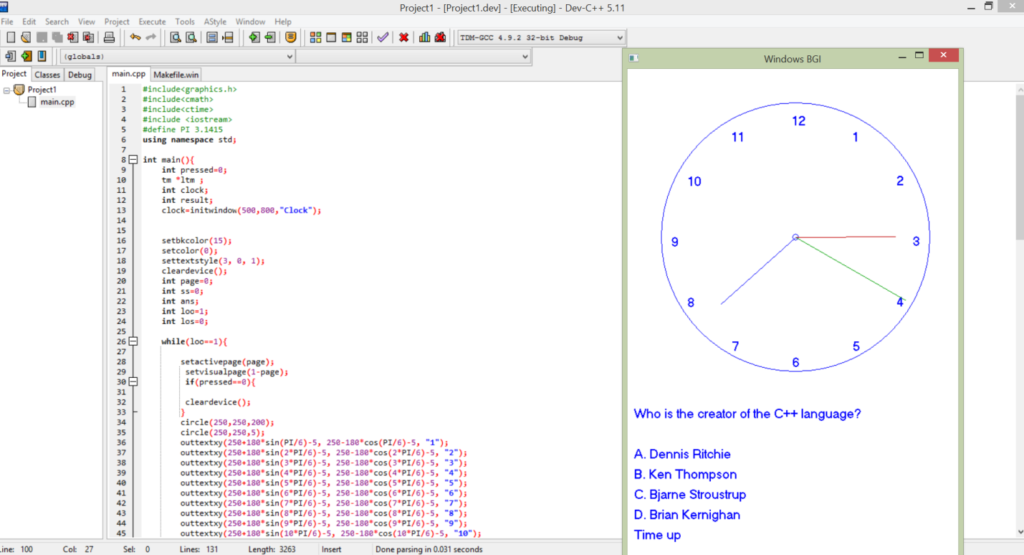
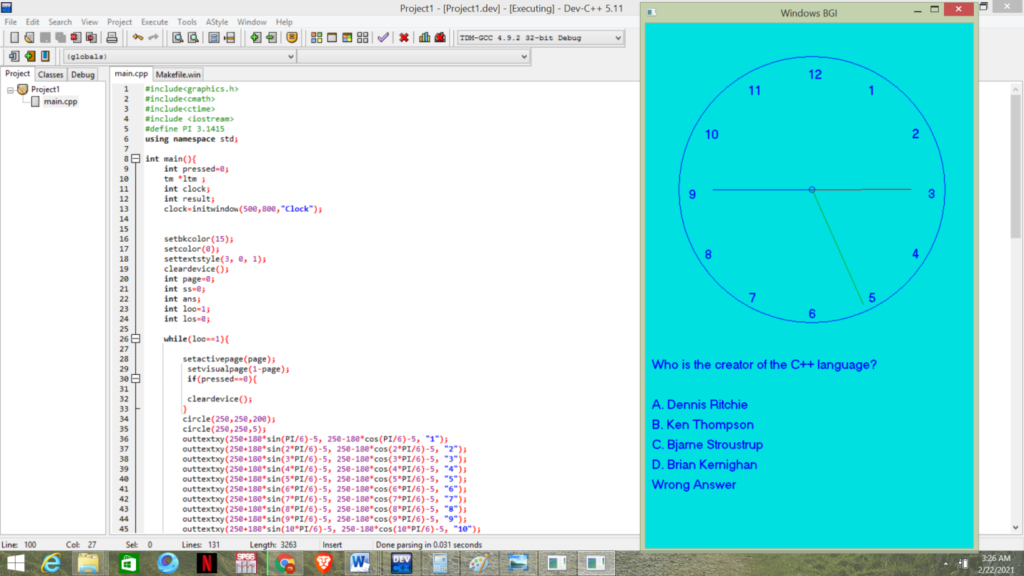
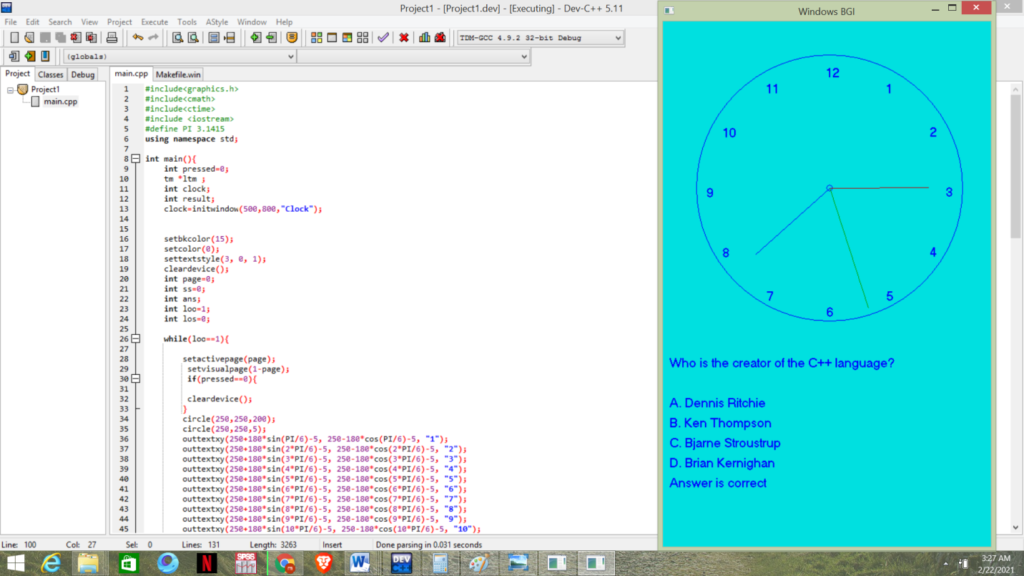
Also read here for more projects