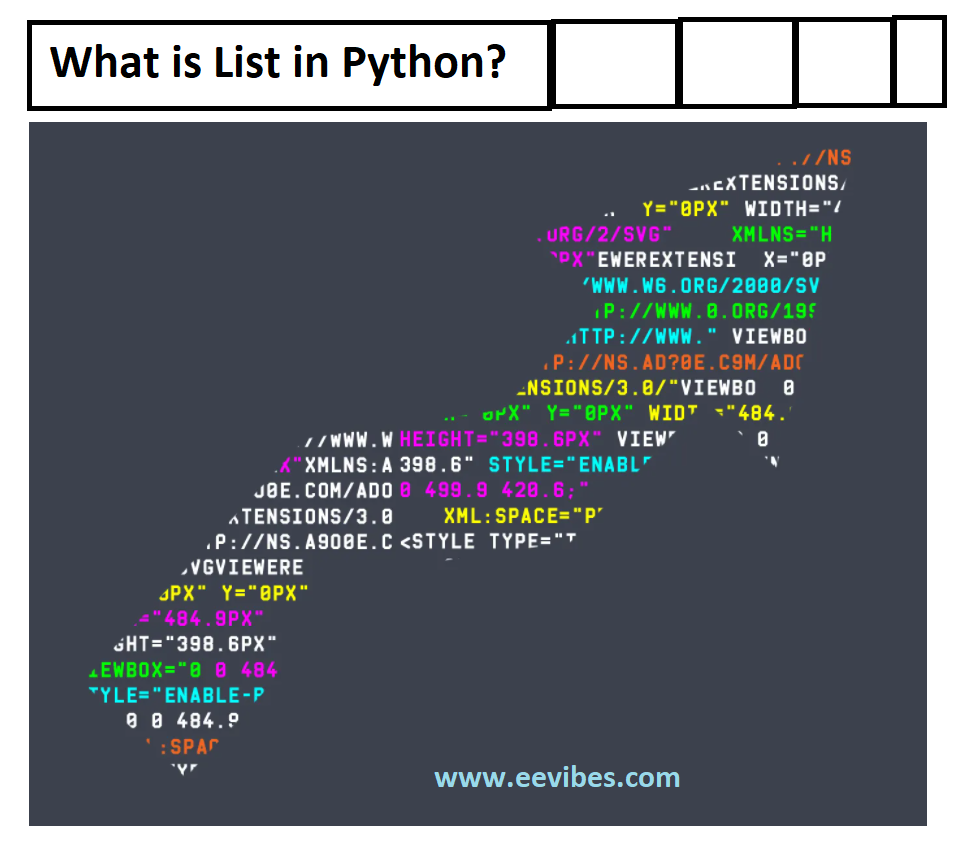
What is a List in Python?
What is a List in Python? List in python language is utilized to store various components in a solitary variable. Records are one of the 4 information types in python language alongside tuple, set, and word reference. List in Python is made by putting components inside the square sections, isolated by commas. A rundown can have quite a few components and it very well may be of numerous information types. Likewise, all the activity of the string is comparatively applied on list information type like cutting, link, and so on Likewise, we can make the settled rundown i.e list containing another rundown.
Utilize the sentence structure while condition with condition as an articulation to emphasize over a square of code until condition assesses to False or isn’t met. Outside of the while circle make a variable to store the current situation inside in the rundown, and addition this worth by one at every emphasis, which will be utilized to file into the rundown. Utilize this methodology on the off chance that each thing in the rundown shouldn’t be gotten to.
List is comparable to clusters in different dialects, with the additional advantage of being dynamic in size. In Python, the rundown is a sort of compartment in Data Structures, which is utilized to store numerous information simultaneously. Dissimilar to Sets, records in Python are requested and have a distinct count.
There are numerous ways of emphasizing over a rundown in Python. How about we see every one of the various ways of repeating over a rundown in Python, and execution examination between them.
Method #1: Using For loop
- Python3
# Python3 code to iterate over a list list = [ 1 , 3 , 5 , 7 , 9 ] # Using for loop for i in list : print (i) |
Output:
1 3 5 7 9
Method #2: For loop and range()
In case we want to use the traditional for loop which iterates from number x to number y.
- Python3
# Python3 code to iterate over a list list = [ 1 , 3 , 5 , 7 , 9 ] # getting length of list length = len ( list ) # Iterating the index # same as 'for i in range(len(list))' for i in range (length): print ( list [i]) |
Output:
1 3 5 7 9
Iterating using the index is not recommended if we can iterate over the elements (as done in Method #1).
Method #3: Using while loop
- Python3
# Python3 code to iterate over a list list = [ 1 , 3 , 5 , 7 , 9 ] # Getting length of list length = len ( list ) i = 0 # Iterating using while loop while i < length: print ( list [i]) i + = 1 |
Output:
1 3 5 7 9
Method #4: Using list comprehension (Possibly the most concrete way).
- Python3
# Python3 code to iterate over a list list = [ 1 , 3 , 5 , 7 , 9 ] # Using list comprehension [ print (i) for i in list ] |
Output:
1 3 5 7 9
Method #5: Using enumerate()
- Python3
# Python3 code to iterate over a list list = [ 1 , 3 , 5 , 7 , 9 ] # Using enumerate() for i, val in enumerate ( list ): print (i, "," ,val) |
Output:
0 , 1 1 , 3 2 , 5 3 , 7 4 , 9
Note: Even method #2 can be used to find the index, but method #1 can’t (Unless an extra variable is incremented every iteration) and method #5 gives a concise representation of this indexing.
Also Read: Returning Multiple values in Java
Method #6: Using numpy
- Python3
# Python program for # iterating over array import numpy as geek # creating an array using # arrange method a = geek.arange( 9 ) # shape array with 3 rows # and 4 columns a = a.reshape( 3 , 3 ) # iterating an array for x in geek.nditer(a): print (x) |
Output:
0 1 2 3 4 5 6 7 8