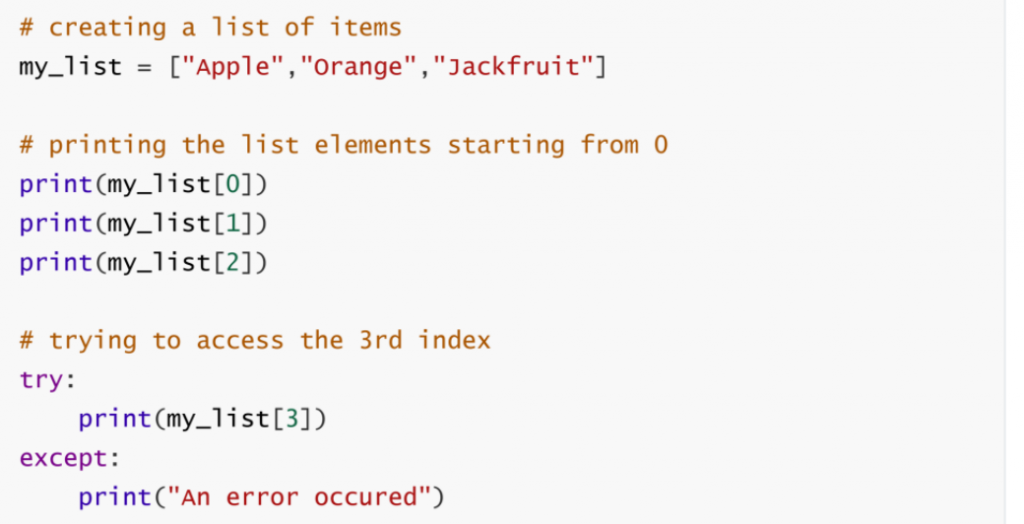
How to remove duplicates from List in Python Programming? You can eliminate copies from a Python utilizing the dict.fromkeys(), which creates a word reference that eliminates any copy esteems. You can likewise change a rundown over to a set. You should change over the word reference or put off into a rundown to see a rundown whose copies have been taken out.
At the point when you’re working with a rundown in Python, there might be a situation where you need to eliminate copies from the rundown. For instance, say you are a gourmet specialist who is blending the old and new menus for your eatery. You might need to eliminate any copies that happen from consolidating the two menus.
This instructional exercise will cover utilizing the dict.fromkeys() technique changing a rundown over to a set. We’ll talk about how to change over the result from these two techniques back into a rundown so you can decipher your information as a rundown.
Table of Contents
Python Remove Duplicates From a List
You can eliminate copies utilizing a Python set or the dict.fromkeys() strategy.
The dict.fromkeys() strategy changes over a rundown into a word reference. Word references can’t contain copy esteems so a word reference with just special qualities is returned by dict.fromkeys().
Sets, similar to word references, can’t contain copy esteems. In the event that we convert a rundown to a set, every one of the copies are taken out.
Eliminate copies from list utilizing Set
To eliminate the copies from a rundown, you can utilize the implicit capacity set(). The strength of set() strategy is that it returns particular components.
We have a rundown : [1,1,2,3,2,2,4,5,6,2,1]. The rundown has many copies which we want to eliminate and get back just the particular components. The rundown is given to the set() worked in work. Later the last rundown is shown utilizing the rundown() worked in work, as displayed in the model beneath.
Technique 1: Naive strategy
In credulous technique, we basically cross the rundown and add the primary event of the component in new rundown and overlook the wide range of various events of that specific component.
# Python 3 code to demonstrate # removing duplicated from list # using naive methods # initializing list test_list = [ 1 , 3 , 5 , 6 , 3 , 5 , 6 , 1 ] print ( "The original list is : " + str (test_list)) # using naive method # to remove duplicated # from list res = [] for i in test_list: if i not in res: res.append(i) # printing list after removal print ( "The list after removing duplicates : " + str (res)) |
Output :
The original list is : [1, 3, 5, 6, 3, 5, 6, 1] The list after removing duplicates : [1, 3, 5, 6]
Technique 2: Using list understanding
This strategy has working like the above technique, yet this is only a joke shorthand of longer strategy finished with the assistance of rundown appreciation.
# Python 3 code to demonstrate # removing duplicated from list # using list comprehension # initializing list test_list = [ 1 , 3 , 5 , 6 , 3 , 5 , 6 , 1 ] print ( "The original list is : " + str (test_list)) # using list comprehension # to remove duplicated # from list res = [] [res.append(x) for x in test_list if x not in res] # printing list after removal print ( "The list after removing duplicates : " + str (res)) |
Output :
The original list is : [1, 3, 5, 6, 3, 5, 6, 1] The list after removing duplicates : [1, 3, 5, 6]
Technique 3: Using set()
This is the most famous way by which the copied are eliminated from the rundown. In any case, the principle and prominent downside of this methodology is that the requesting of the component is lost in this specific technique.
# Python 3 code to demonstrate # removing duplicated from list # using set() # initializing list test_list = [ 1 , 5 , 3 , 6 , 3 , 5 , 6 , 1 ] print ( "The original list is : " + str (test_list)) # using set() # to remove duplicated # from list test_list = list ( set (test_list)) # printing list after removal # distorted ordering print ( "The list after removing duplicates : " + str (test_list)) |
Output :
The original list is : [1, 5, 3, 6, 3, 5, 6, 1] The list after removing duplicates : [1, 3, 5, 6]
Technique 4: Using list understanding + count()
list perception combined with count capacity can likewise accomplish this errand. It fundamentally searches for currently happened components and skips adding them. It saves the rundown requesting.
# Python 3 code to demonstrate # removing duplicated from list # using list comprehension + enumerate() # initializing list test_list = [ 1 , 5 , 3 , 6 , 3 , 5 , 6 , 1 ] print ( "The original list is : " + str (test_list)) # using list comprehension + enumerate() # to remove duplicated # from list res = [i for n, i in enumerate (test_list) if i not in test_list[:n]] # printing list after removal print ( "The list after removing duplicates : " + str (res)) |
Output :
The original list is : [1, 5, 3, 6, 3, 5, 6, 1] The list after removing duplicates : [1, 5, 3, 6]
Technique 5: Using collections.OrderedDict.fromkeys()
This is quickest strategy to accomplish the specific errand. It first eliminates the copies and returns a word reference which must be changed over to list. This functions admirably if there should arise an occurrence of strings moreover.
# Python 3 code to demonstrate # removing duplicated from list # using collections.OrderedDict.fromkeys() from collections import OrderedDict # initializing list test_list = [ 1 , 5 , 3 , 6 , 3 , 5 , 6 , 1 ] print ( "The original list is : " + str (test_list)) # using collections.OrderedDict.fromkeys() # to remove duplicated # from list res = list (OrderedDict.fromkeys(test_list)) # printing list after removal print ( "The list after removing duplicates : " + str (res)) |
Output :
The original list is : [1, 5, 3, 6, 3, 5, 6, 1] The list after removing duplicates : [1, 5, 3, 6]
Also Read: How to set checkbox size in HTML/CSS?