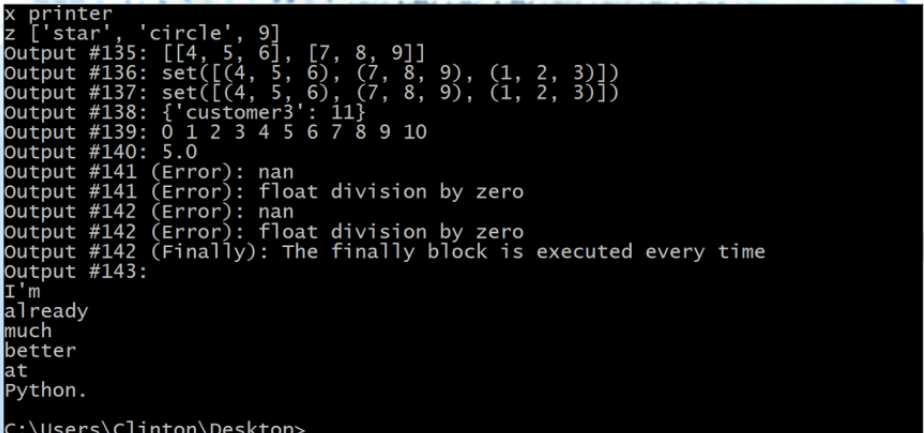
How to Sort Python Dictionaries by Key or Value? A word reference in Python is an assortment of things that stores information as key-esteem sets. In Python 3.7 and later forms, word references are arranged by the request for thing addition. In prior variants, they were unordered.
We can sort a word reference with the assistance of a for circle. To begin with, we utilize the arranged() capacity to arrange the upsides of the word reference. We then, at that point, circle through the arranged qualities, tracking down the keys for each worth. We add these keys-esteem sets in the arranged request into another word reference.
We can sort records, tuples, strings, and other iterable articles in python since they are totally requested items. All things considered, as of python 3.7, word references recollect the request for things embedded also. Hence we are likewise ready to sort word references utilizing python’s inherent arranged() work. Very much like with other iterables, we can sort word references in view of various standards relying upon the critical contention of the arranged() work.
Python programming language comprises of crude information types like rundown, word reference, set, tuple and so forth It additionally accompanies an assortments module which has particular information structures like Chain Map, deque and so forth It turns out to be not difficult to work with these information types as they comprise of capacities which makes the code proficient. Sort work is one such capacity for a word reference in python. In this article we will examine how we can sort a word reference. Following are the ideas examined in this blog:
Problem Statement – Here are the major tasks that are needed to be performed.
- Create a dictionary and display its keys alphabetically.
- Display both the keys and values sorted in alphabetical order by the key.
- Same as part (ii), but sorted in alphabetical order by the value.
Approach
Load the Dictionary and perform the following operations:
- First, sort the keys alphabetically using key_value.iterkeys() function.
- Second, sort the keys alphabetically using sorted (key_value) function & print the value corresponding to it.
- Third, sort the values alphabetically using key_value.iteritems(), key = lambda (k, v) : (v, k))
Let’s try performing the above-mentioned tasks:
Displaying the Keys Alphabetically:
Examples:
Input: key_value[2] = '64' key_value[1] = '69' key_value[4] = '23' key_value[5] = '65' key_value[6] = '34' key_value[3] = '76' Output: 1 2 3 4 5 6
Program:
- Python3
# Function calling def dictionairy(): # Declare hash function key_value = {} # Initializing value key_value[ 2 ] = 56 key_value[ 1 ] = 2 key_value[ 5 ] = 12 key_value[ 4 ] = 24 key_value[ 6 ] = 18 key_value[ 3 ] = 323 print ( "Task 1:-\n" ) print ( "Keys are" ) # iterkeys() returns an iterator over the # dictionary’s keys. for i in sorted (key_value.keys()) : print (i, end = " " ) def main(): # function calling dictionairy() # Main function calling if __name__ = = "__main__" : main() |
Output:
Task 1:- Keys are 1 2 3 4 5 6
Sorting the Keys and Values in Alphabetical Order using the Key.
Examples:
Input: key_value[2] = '64' key_value[1] = '69' key_value[4] = '23' key_value[5] = '65' key_value[6] = '34' key_value[3] = '76' Output: (1, 69) (2, 64) (3, 76) (4, 23) (5, 65) (6, 34)
Program:
- Python3
# function calling def dictionairy(): # Declaring the hash function key_value = {} # Initialize value key_value[ 2 ] = 56 key_value[ 1 ] = 2 key_value[ 5 ] = 12 key_value[ 4 ] = 24 key_value[ 6 ] = 18 key_value[ 3 ] = 323 print ( "Task 2:-\nKeys and Values sorted in" , "alphabetical order by the key " ) # sorted(key_value) returns an iterator over the # Dictionary’s value sorted in keys. for i in sorted (key_value) : print ((i, key_value[i]), end = " " ) def main(): # function calling dictionairy() # main function calling if __name__ = = "__main__" : main() |
Output:
Task 2:- Keys and Values sorted in alphabetical order by the key (1, 2) (2, 56) (3, 323) (4, 24) (5, 12) (6, 18)
Sorting the Keys and Values in alphabetical using the value
Examples:
Input: key_value[2] = '64' key_value[1] = '69' key_value[4] = '23' key_value[5] = '65' key_value[6] = '34' key_value[3] = '76' Output: (4, 23), (6, 34), (2, 64), (5, 65), (1, 69), (3, 76)
Program:
- Python3
# Function calling def dictionairy(): # Declaring hash function key_value = {} # Initializing the value key_value[ 2 ] = 56 key_value[ 1 ] = 2 key_value[ 5 ] = 12 key_value[ 4 ] = 24 key_value[ 6 ] = 18 key_value[ 3 ] = 323 print ( "Task 3:-\nKeys and Values sorted" , "in alphabetical order by the value" ) # Note that it will sort in lexicographical order # For mathematical way, change it to float print ( sorted (key_value.items(), key = lambda kv:(kv[ 1 ], kv[ 0 ]))) def main(): # function calling dictionairy() # main function calling if __name__ = = "__main__" : main() |
Output:
Task 3:- Keys and Values sorted in alphabetical order by the value [(1, 2), (5, 12), (6, 18), (4, 24), (2, 56), (3, 323)]
Sort the dictionary by key
Program:
- Python3
# Creates a sorted dictionary (sorted by key) from collections import OrderedDict dict = { 'ravi' : '10' , 'rajnish' : '9' , 'sanjeev' : '15' , 'yash' : '2' , 'suraj' : '32' } dict1 = OrderedDict( sorted ( dict .items())) print (dict1) |