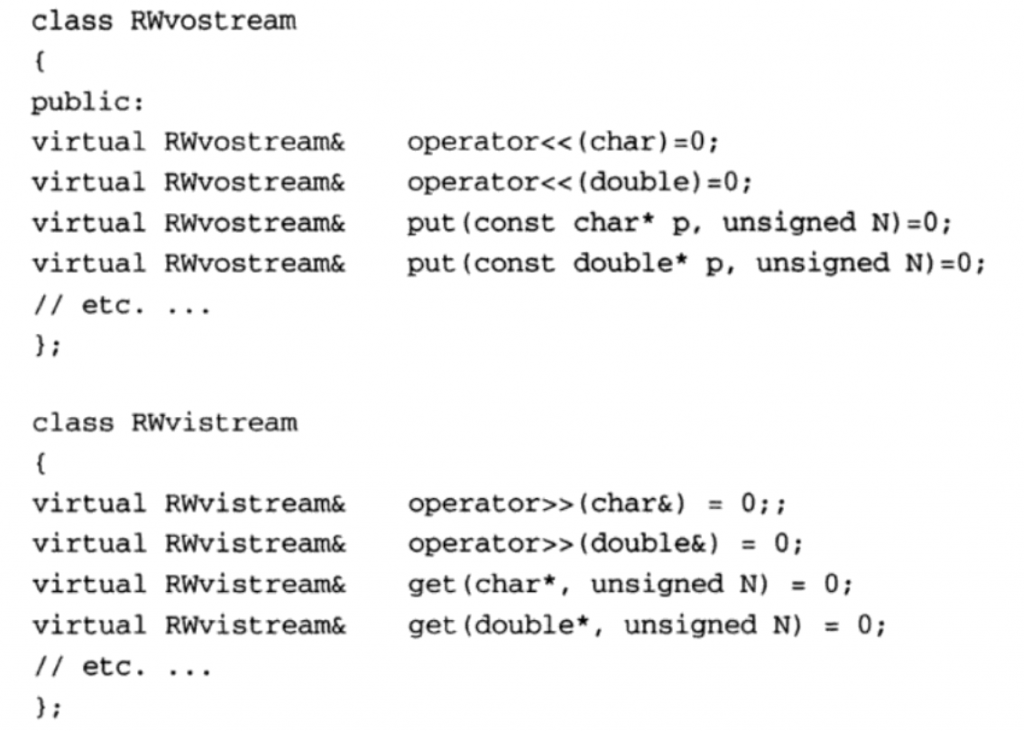
What are the different methods for reversing a string in C/C++? Switching a string alludes to the procedure on a string, by which the grouping of characters in it gets turned around. For instance, consider ‘str’ contains a string “JournalDev” in it. After the inversion activity happens on the string ‘str’, the substance ought to get switched. Thus now, ‘str’ ought to contain the string “veDlanruoJ”. Presently let us perceive how we can play out this opposite procedure on C++ strings utilizing different strategies.
Switching a string alludes to the procedure on a string, by which the grouping of characters in it gets turned around. For instance, consider ‘str’ contains a string “JournalDev” in it. After the inversion activity happens on the string ‘str’, the substance ought to get switched. Thus now, ‘str’ ought to contain the string “veDlanruoJ”. Presently let us perceive how we can play out this opposite procedure on C++ strings utilizing different strategies.
Example
User-defined reverse() function −
#include <bits/stdc++.h> using namespace std; //function to reverse given string void reverse_str(string& str){ int n = str.length(); for (int i = 0; i < n / 2; i++) swap(str[i], str[n - i - 1]); } int main(){ string str = "tutorialspoint"; reverse_str(str); cout << str; return 0; }
Using in-built reverse() function −
#include <bits/stdc++.h> using namespace std; int main(){ string str = "tutorialspoint"; reverse(str.begin(), str.end()); cout << str; return 0; }
Printing reverse of a given string−
#include <bits/stdc++.h> using namespace std; void reverse(string str){ for (int i=str.length()-1; i>=0; i--) cout << str[i]; } int main(void){ string s = "tutorialspoint"; reverse(s); return (0); }
Output
eevibes
Different Methods to Reverse a String in C/C++
1- Using reverse()
reverse()
method available in the algorithm header file can reverse a string in-place without writing any boilerplate code.
All we need to do is to pass the beginning and end iterator as arguments to the particular method.
Here is an example in C++, demonstrating the same:
#include <iostream>
#include<algorithm>
using namespace std;
int main() {
string str = "Hello world!";
reverse(str.begin(), str.end());
cout << str;
return 0;
}
Output:
!dlrow olleH
2- Using Loop
We can also reverse a string using ‘for’ or ‘while’ loop without any built-in funtion.
The idea is to iterate through the characters of the input string in reverse order and concatenate them to a new string.
To do this, we have to follow the following steps:
- Input a string.
- for loop from
str.length()
to0
in decrementing order.- Concatenate each character to the reverse string i.e.,
rev = rev + str[i]
.
- Concatenate each character to the reverse string i.e.,
- Output the reverse string.
Here is the implementation of the steps in C++:
#include <iostream>
using namespace std;
int main()
{
//Input string
string str = "code";
//Initialize reverse with null string value
string rev = "";
//Iterate input string in revrese order
for(int i=str.length()-1; i>=0; i--){
//concatenate characters to the reverse
rev = rev + str[i];
}
cout << rev;
return 0;
}
Output:
edoc
3- Using Recusion
We can also reverse a string using recursion in C++. The idea is to extract the characters on each recursive call and reassign them to the string in reverse order during the traceback process.
Here is the implementation of the idea in C++:
#include <iostream>
using namespace std;
//recursive function
void reverse(string &str, int i){
//Base condition - when index exceeds string length
if(i == str.length())
return;
//extract character
char ch = str[i];
//recusively call for next character
reverse(str, i+1);
//reassign in reverse order
str[str.length()-i-1] = ch;
}
int main()
{
//Input string
string str = "Pencil Programmer";
//start recirsive funtion from first index
reverse(str, 0);
//output the string
cout << str;
return 0;
}
Output:
remmargorP licneP
Also Read: How to Split a String in Golang?