Table of Contents
Introduction to random numbers in c++
How to use rand() function in C++? How does it generate random number? An interesting programming application in C++ is the random number generation. Different game playing programs can be developed using its concept specially the card game. “The element of chance” can be introduced using the built in function rand() that is defined in <stdlib>. It can generate the values between 0 and RAND_MAX. RAND_MAX is the symbolic constant that is defined in <stdlib>. The value of this symbolic constant depends on your window system .i.e., for 16 bit system it is 32767. Every number between 0 and RAND_MAX has equal chance of occurrence each time the rand() function is called.
Scaling of random numbers
Obviously the range of values generated by this function vary than the values required by the application. For example, a program that involves rolling a six face dice needs values between 1 and 6 only. Similarly, tossing a coin only needs head “0” or tail “1”. For generating the values between the desired range the modulus (%) operator is used. So in case of dice the following syntax is used
rand()%6;
This process is called scaling and the constant 6 is called scaling factor. Then we add 1 for shifting the range of numbers.
Example
consider a case where a dice is rolled 2000 times and we are interested in calculating how many times all faces (1,2,3,4,5,6) appear .
Solution
void main()
{
clrscr();
int f1=0,f2=0,f3=0,f4=0,f5=0,f6=0;
int face;
for(int roll=1; roll<=2000;roll++)
face=1+rand()%6;
switch(face)
{
case 1:
++f1;
break;
case 2:
++f2;
break;
case 3:
++f3;
break;
case 4:
++f4;
break;
case 5:
++f5;
break;
case 6:
++f6;
break;
default: cout<<“Not valid”<<endl;
}
}
cout<<“frequency of 1″<<f1<<endl;
cout<<“frequency of 2″<<f2<<endl;
cout<<“frequency of 3″<<f3<<endl;
cout<<“frequency of 4″<<f4<<endl;
cout<<“frequency of 5″<<f5<<endl;
cout<<“frequency of 6″<<f6<<endl;
getch();
}
Randomizing the random number Generator.
If the program is executed then the output remains same. Means the fixed random numbers are generated each time, it is executed. Then how is it possible to call the rand function generates the random numbers?
It actually generates the “pseudo random numbers“. If the rand function is called repeatedly then it will produce the sequence of random numbers. But that sequence of numbers will repeat each time it is executed. In order to produce different sequence of random numbers srand() function is used. This approach is called “randomizing the random numbers”. srand() function is used for forcing the rand() function to generate the different sequence of random numbers each time it is executed. The input to srand() is an unsigned integer.
Example
void main()
{
clrscr();
unsigned putvalue;
cin>>putvalue;
srand(putvalue);
for(int i=0;i<=5;i++)
{
cout<<setw(10)<<(1+rand()%6);
if(i%4==0)
cout<<endl;
}
}
now if the above program is executed then each time it will generate random numbers for different values of “putlvalue”. But if the same value is used for “putvalue” then again the output is same. This problem can be overcome by using the time function.
srand(time(0));
The above statement will cause the computer to take value of “putvalue” from its clock.
How to use rand() function in C++?
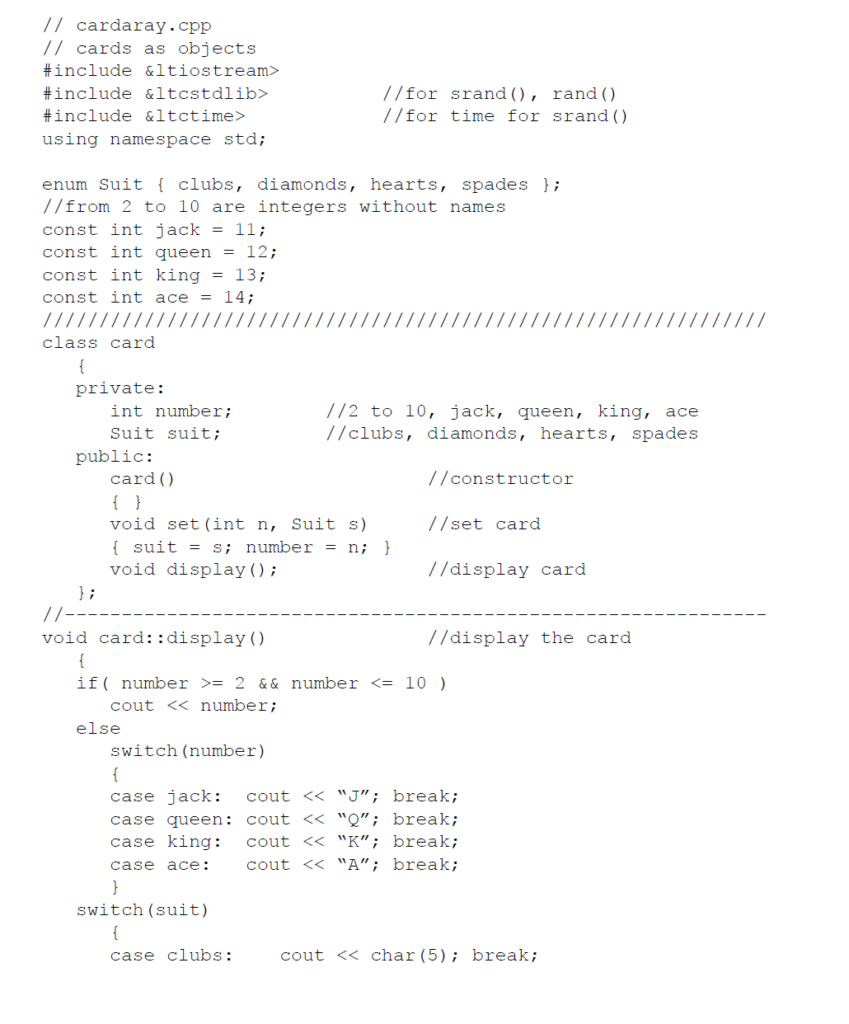
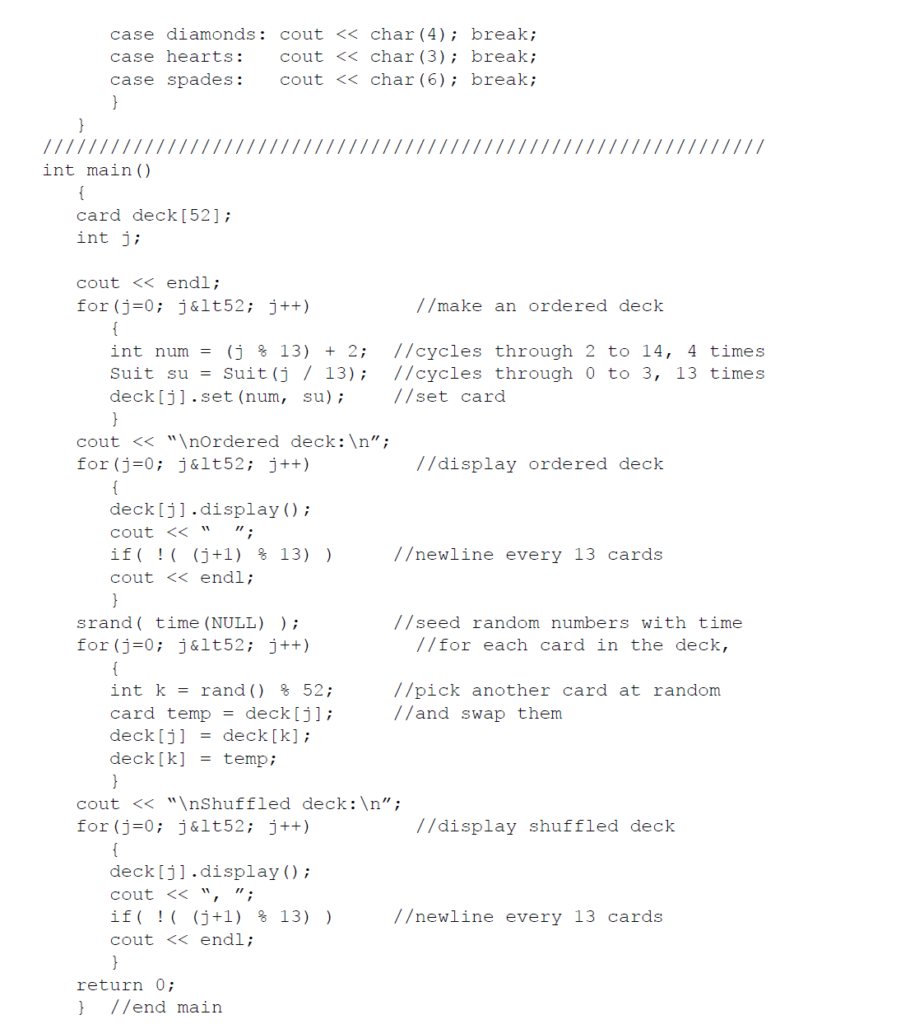
Also read here:
https://eevibes.com/recursion-in-c-programming/
One thought on “How to use rand() function in C++?”