Table of Contents
Introduction
What are the strings in C++?. How do we use them? Strings in C++ are treated as array of characters. For example the phrase “happiness is the state of mind” and “humanity comes first” are both the strings of characters. These phrases are stored in a consecutive group of memory locations and are terminated at a special character, called the “null character \0”. The ASCII code for null character is 0. The phrases defined above are stored as:
H | a | p | p | i | n | e | s | s | i | s | t | h | e | s | t | a | t | e | o | f | m | i | n | d | \0 |
H | u | m | a | n | i | t | y | c | o | m | e | s | f | i | r | s | t | \0 |
If we define the string length to be of size 10, then only 9 characters can be stored as the last character space is for null character.
String Constant :
String constant is actually a string enclosed in double quotes (“”). For example “my first blog”. When a string constant is printed, its memory address is actually accessed. When we write down the statement cout<<“say hello”; then all characters are printed until the null character is reached.
Storing strings in arrays :
when we start storing the strings in arrays the problem that may arise is shown in the following example.
Example
void main()
{
const int length=30;
char str[length];
cin>>str;
}
Now lets say user enters “hello” then this is ok. But what if a user wants to enter “hello how are you?” then this cin>> command treats the “space character as terminating or null character. So, when we use cout<<str; command only hello is displayed and the rest of the string characters are skipped.
Reading embedded blanks
In order to overcome the above mentioned problem, we need to read the embedded blanks. For this there is a builtin function cin.getline(array_name, size). So this is how it works.
void main()
{
const int sizee=30;
char str[sizee];
cout<<“enter the string”<<endl;
cin.getline(str,sizee);
cout<<“the string is”<<str<<endl;
}
now the whole string will be printed. What are the strings and how to do implementation of strings in C++ answer is given above.
Copying the strings
There are two ways to copy one string into another. The first way is by copying one by one character which is little harder while the second way is to use the builtin function strcpy(destination,source). This function is defined in header file string.h.
First Approach
void main()
{
char str1[]=”hello world”;
const int sizee=30;
char str2[sizee];
for(int i=0;i<strlen(str1);i++)
str2[i]=str1[i];
str2[i]=’\0′;
cout<<str2;
}
Second Approach
void main()
{
char str1[]=”hello world”;
cont int sizee=30;
char str[sizee];
strcpy(str2,str1);
cout<<str2;
}
Arrays of Strings
There are many cases where we see arrays of strings. It is a very useful construction. Here is an example that puts days of week in an array.
Question
Write down a program for putting days ok week in an array using the concept of arrays of strings.
Solution
#include<iostream.h>
#include<conio.h>
int main()
{
const int days = 7;
const int max = 10;
char star[days][max] = { “Sunday”, “Monday”, “Tuesday”,
“Wednesday”, “Thursday”,
“Friday”, “Saturday” };
for(int i=0; i<tdays; i++) //display every string
cout << star[i] << endl;
return 0;
}
Since a string is a cluster, the facts must confirm that star—a variety of strings—is actually a two-dimensional exhibit. The primary element of this cluster, days, tells the number of strings are in the exhibit. The subsequent measurement, max, indicates the most extreme length of the strings (9 characters for “Wednesday” in addition to the ending invalid makes 10).
This is how a 2-D array is stored
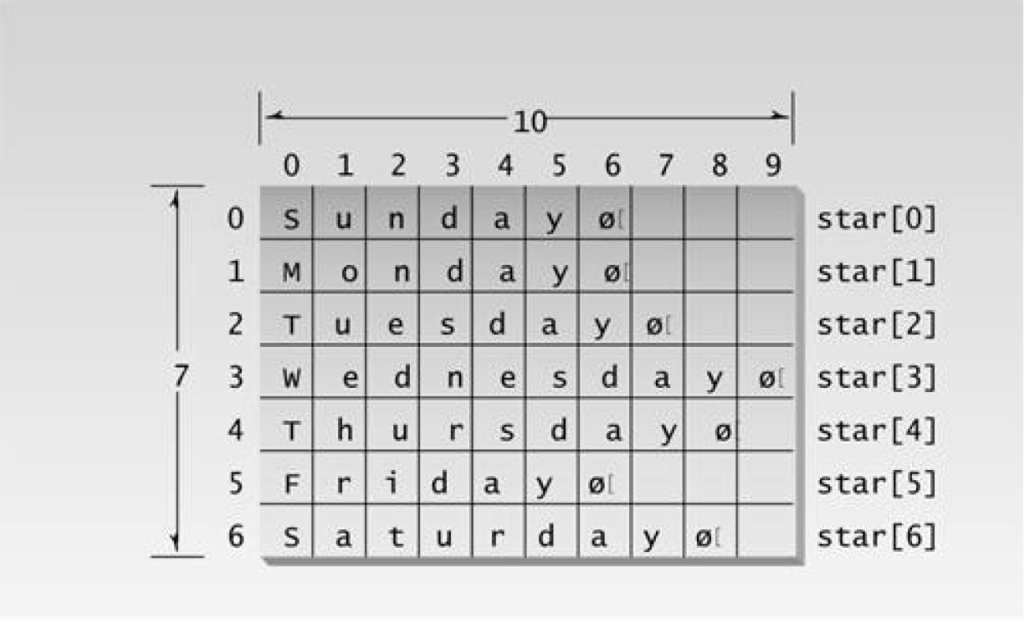
A User-Defined String Type
There are a few issues with C-strings as they are regularly utilized in C++. For a certain something, you can’t utilize the totally sensible articulation to set one string equivalent to another. (In certain dialects, similar to BASIC, this is totally okay.) The Standard C++ string class we’ll inspect in the following segment will deal with this issue, yet for the second we should check whether we can utilize object-situated innovation to tackle the difficult ourselves. Making our own string class will give us an understanding into speaking to strings as objects of a class, which will enlighten the activity of of Standard C++ string class. On the off chance that we characterize our own string type, utilizing a C++ class, we can utilize task proclamations. (Numerous other C-string tasks, for example, link, can be streamlined this route also, however we’ll need to stand by until Part 8, “Administrator Overloading,” to perceive how this is finished.) The STROBJ program makes a class called String. (Try not to confound this hand crafted class String with the Standard C++ worked in class string, which has a lowercase ‘s’.) Here’s the example of listing:
#include<iostream.h>
#include<string.h>
#include<conio.h>
class String
{
private:
enum { siz = 80; };
char str[siz];
public:
String()
{ str[0] = ‘\0’; }
String( char s[] )
{ strcpy(str, s); }
void display()
{ cout << str; }
void concat(String s2)
{
if( strlen(str)+strlen(s2.str) < SZ )
strcat(str, s2.str);
else
cout << “\nString too long”;
}
};
int main()
{
String s1(“Happy holidays ”);
String s2 = “enjoy your time”;
String s3;
cout << “\ns1=”; s1.display();
cout << “\ns2=”; s2.display();
cout << “\ns3=”; s3.display();
s3 = s1; //assignment
cout << “\ns3=”; s3.display();
s3.concat(s2);
cout << “\ns3=”; s3.display();
cout << endl;
return 0;
}
Also read here
https://eevibes.com/single-dimension-arrays-and-searching-algorithms/
What are the single dimension arrays? How many searching algorithms are there for searching a key?