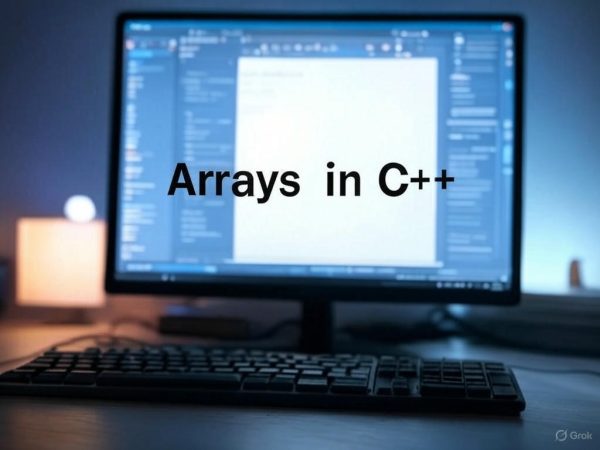
Introduction to arrays
What are the single dimension arrays? Arrays are the consecutive group of memory locations that have same name of same data types. In programming, arrays are used to “group” the data items of same type. SO rather than declaring multiple data items of same types we use the concept of arrays. The syntax for declaring the arrays is:
int newarray[10];
the array with name “newarray” is declared and it has the capacity of 10 elements which means array size is 10. The array index starts from 0 and ends on arraysize-1. In this case the first element of array is indexed as newarray[0] and the last one is newarray[9]. If we want to access any element of an array we need to provide two things: the first one is array name and the second one is array index. So, if we want to access the third element of array named newarray, then we will write as newarray[2].
Initializing Array
We can also initialize the array elements like: int newarray[10]={2,4,6,7,8,3,9,0,5}; so they will be filled as:

Another way to initialize the array elements is using for loop. For example
for(int i=0;i<10;i++)
{
cin>>newarray[i];
}
Some operation that we can perform on arrays
Lets do how can we play with array elements.
Example 1: Program for finding the largest element of an array
Write a program to find the maximum element of the array.
void main()
{
int n[10];
int max=0;
for(int i=0;i<10;i++)
{
cin>>n[i];
}
for(int j=0;j<10;j++)
{
if(n[j]>max)
max=n[j];
}
cout<<“maximum element of array is:”<<max;
getche();
}
Example 2: Program for finding the smallest element of an array
Similarly, we can also find the smallest element of an array as done below
int min=0;
for(int k=0;k<10;k++)
{
if(n[k]<min)
min=n[k];
}
cout<<“minimum element is”<<min;
}
Now try to solve some question
How to find the sum of array elements?
Exercise 1
Write down a C++ program to find either the pressed key is present or not in an array.
Solution
void main()
{
int abc[10];
int key;
cin>>key;
for(int i=0; i<10;i++)
{
cin>>abc[i];
}
for(int j=0;j<10;j++)
{
if(key==abc[j])
cout<<“key is found”;
break;
}
cout<<“key not found”;
}
Exercise 2
Twenty students were asked to rate on a scale of 1 to 5 the quality of the food in the student cafeteria, with 1 being “awful” and 5 being “excellent.” Place the 20 responses in an integer array and determine the frequency of each rating. Your output should look like the following table.
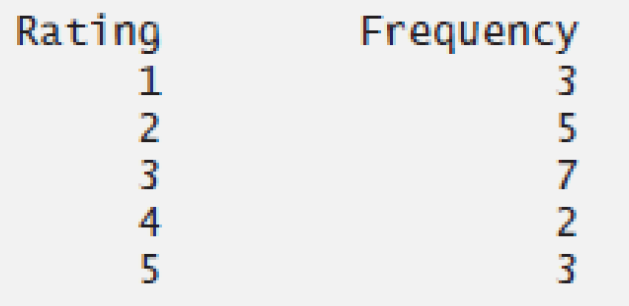
Solution:
#include<iostream.h>
#include<conio.h>
void perofromance(int feedback[],int size)
{
int f1=0,f2=0,f3=0,f4=0,f5=0;
for(int j=0;j<size;j++)
{
if(feedback[j]==1)
f1++;
else
if(feedback[j]==2)
f2++;
else
if(feedback[j]==3)
f3++;
else
if(feedback[j]==4)
f4++;
else
f5++;
}
cout<<“rating”<<” “<<“frequency”<<endl;
cout<<“1″<<” “<<f1<<endl;
cout<<“2″<<” “<<f2<<endl;
cout<<“3″<<” “<<f3<<endl;
cout<<“4″<<” “<<f4<<endl;
cout<<“5″<<” “<<f5<<endl;
}
void main()
{
clrscr();
int feedback[20];
for(int i=0;i<20;i++) { cin>>feedback[i];
}
performance(feedback[],20);
getch();
}
Exercise 3
Consider a professor wants to graph the number of grades in each of several categories to visualize the grade
distribution.Suppose the grades were 87, 68, 94, 100, 83, 78, 85, 91, 76 and 87. There was one grade of 100,
two grades in the 90s, four grades in the 80s, two grades in the 70s, one grade in the 60s and no grades below
60. Your output should look like the following.
Solution:
#include<iostream.h>
#include<conio.h>
void display(int n)
{
for(int i=0;i<n;i++)
cout<<“*”<<endl;
}
void compute(int grades[], int n)
{
int record[10]={0,0,0,0,0,0,0,0,0,0};
for(int i=0;i<n;i++)
{
if(grades[i]>=0&& grades[i]<=9)
record[0]++;
else
if(grades[i]>=10&& grades[i]<=19)
record[1]++;
else
if(grades[i]>=20&& grades[i]<=29)
record[2]++;
else
if(grades[i]>=30&& grades[i]<=39)
record[3]++;
else
if(grades[i]>=40&& grades[i]<=49)
record[4]++;
else
if(grades[i]>=50&& grades[i]<=59)
record[5]++;
else
if(grades[i]>=60&& grades[i]<=69)
record[6]++;
else
if(grades[i]>=70&& grades[i]<=79)
record[7]++;
else
if(grades[i]>=80&& grades[i]<=89)
record[8]++;
else
record[9]++;
}
cout<<“grades:”<<” “<<“distribution”<<endl;
cout<<“0-9:”<<” “<<endl;
display(record[0]);
cout<<“10-19:”<<” “<<endl;
display(record[1]);
cout<<“20-29:”<<” “<<endl;
display(record[2]);
cout<<“30-39:”<<” “<<endl;
display(record[3]);
cout<<“40-49:”<<” “<<endl;
display(record[4]);
cout<<“50-59:”<<” “<<endl;
display(record[5]);
cout<<“60-69:”<<” “<<endl;
display(record[6]);
cout<<“70-79:”<<” “<<endl;
display(record[7]);
cout<<“80-89:”<<” “<<endl;
display(record[8]);
cout<<“90-100:”<<” “<<endl;
display(record[9]);
}
void main()
{
clrscr();
int grades[10];
for(int m=0;m<10;m++)
{
cin>>grades[m];
}
compute(grades,10);
getch();
}
watch here for video lecture
Also read here
3 thoughts on “What are the Arrays in C++ Programming?”