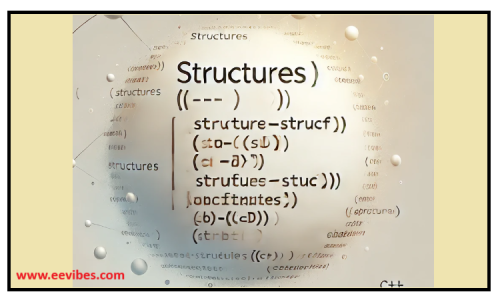
Introduction to Structures in C++
In programming, we encounter different types of variables. Some of them are of int type, some are floats, and others are double or character. Such types of variables represent one type of information e.g., length, marks, model name or model type. But there are certain kind of information that need more detail for its representation. For example a school building has rooms, windows, no. of chairs, specific number of students and so on. So such kind of variables or entities need to be binded in more suitable ways and that is structure in C++.
Structures represent the collection of variables of different data types. These variables are called the members of structures. They are building blocks for understanding the concept of classes and objects. Structures combine different data items while classes combine both the data items and functions.
How to Define a Structure?
Here is a simple example of how to define a structure in C++. Lets define a structure for a school building that has the capacity of fixed number of class rooms, no. of chair in each class, and class names associated to each room.
Example
Struct school
{
int rooms;
int chaircount;
char roomname;
};
so we have defined here a structure with reserve keyword “struct” named as “school” and it has three members. This is merely a blueprint of the structure and this declaration does not occupy any space in memory of computer system.
The next step is to define a variable of structure type so we can access the members of the structure through it. By accessing we mean we can initialize those members and then can print their values. We can also perform different kind of functions on them.
Structure Variable
A structure variable is defined by using the structure name as its “type” and then we can name it any. For the above structure school we can define a variable s1. So, school s1 is the structure variable. A structure can have more than one variables.
school s1;
school s2;
Accessing Structure Members using Structure Variables.
Lets see how can we use these variables now for initializing the members of a structure.
- The first approach is to directly initialize the members using structure variable. e.g.,
school s1={ 5, 100, ‘seminar hall’};
This statement will automatically initialize each member in this particular order. It means rooms=5, chaircount=100, and roomname= seminar hall. Another way to initialize the memebrs is by using the dot operator. The dot operator works like that:
cin>>s2.rooms;
cin>>s2.chaircount;
cin>>s2.roomname
We can also copy the value of one structure variable into another. For example s1=s2. This statement will be replicating the values of structure members.
Example
Create a structure for representing the distance in form of two parameters: feet and inches.
struct distance
{
int feet;
float inches;
};
void main()
{
distance d1,d3;
d2={1,7};
cin>>d1.feet;
cin>>d1.inches;
d3.inches=d1.inches+d2.inches;
if(d3.inches>=12)
{
d3.inches=d3.inches-12;
d3.feet++;
}
d3.feet+=d1.feet+d2.feet;
cout<<“d3 feet”<<d3.feet<<“d3 inches”<<d3.inches;
}
Question #1
A phone number, such as (212) 767-8900, can be thought of as having three parts: the area code (212), the exchange (767), and the number (8900). Write a program that uses a structure to store these three parts of a phone number separately. Create two structure variables of type phone. Initialize one, and have the user input a number for the other one. Then display both numbers.
Solution
struct phone
{
int areacode;
int exchange;
int numb;
};
void main()
{
phone p1={123,456,789};
phone p2;
cin>>p2.areacode;
cin>>p2.exchange;
cin>>p2.numb;
cout<<“the phone number is”<<p1.areacode<<p1.exchange<<p1.numb<<endl;;
cout<<“the phone number is”<<p2.areacode<<p2.exchange<<p2.numb;
}
Question # 2
There is another case for designing a structure of a plaza where each shop has its name, its rent and total sales of a month. You will be designing two structures named as plaza and shop. The plaza will have attributes total sales, total rent, and profit. Total profit can be calculated as the difference of total sales and total rent.
Solution:
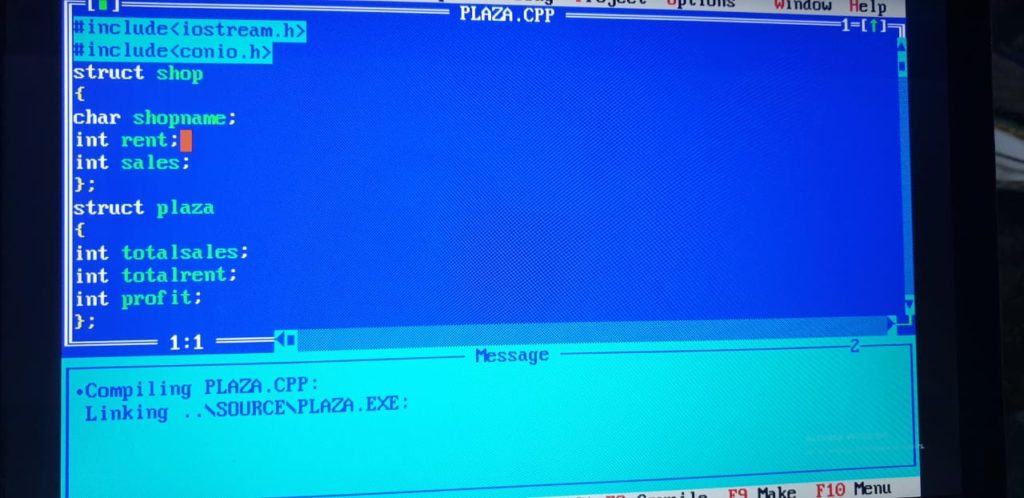
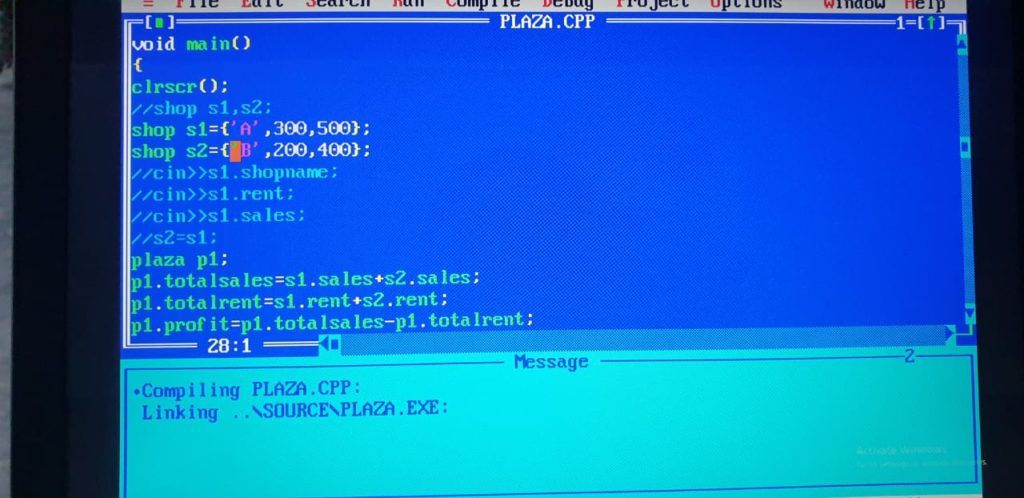
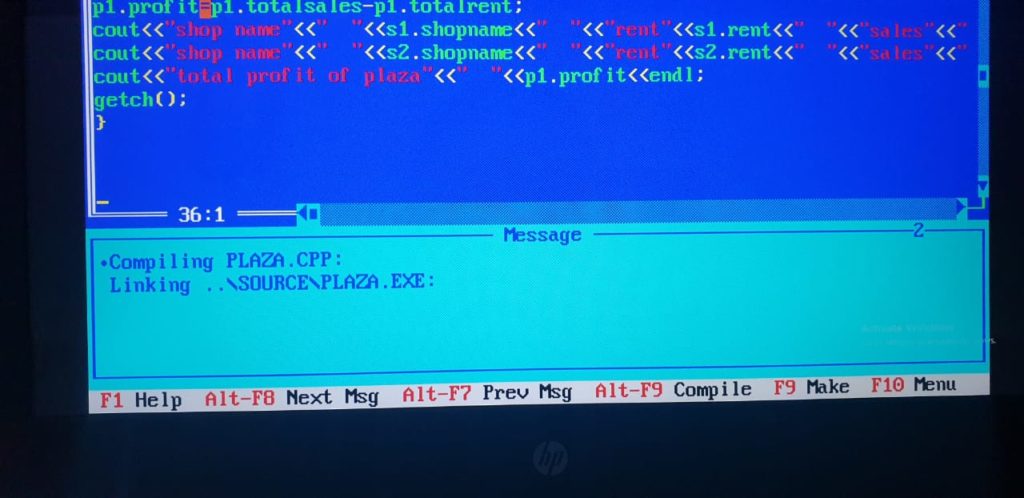
Here is the video lecture that explains structures with an example
Structures within Structures
We can also use the concept of structures within structures. Here the features of a room like length and width can be measured in feet and inches. Thus the dimensions of a room can be represented using the concept of nested structures.
Example
struct distance
{
int feet;
int inches;
};
struct room
{
distance length;
distance width;
};
void main()
{
clrscr();
room dining;
dining.length.feet=15;
dining.length.inches=6;
dining.width.feet=13;
dining.width.inches=9;
float l=dining.length.feet+dining.length.inches/12;
float w=dining.width.feet+dining.width.inches/12;
cout<<“dining room area is <<l*w<<“square feet”<<endl;
}
Arrays of Structures:
It is also possible to define an array with the structure name. The purpose of this approach is to have access to the structure variables. In simple words, this will help to assign values to structure variables through arrays. Hence structure variables act as array elements. Lets consider an example and see how arrays of structures can be defined.
Example:
Consider a car company that manufactures different types of cars and the owner wants to maintain the record of 5 cars name, their model number, their color, and cost. This can be done using the concept of arrays of structures.
solution:
struct company
{
char modelname[20];
int modelno;
int cost
char color[20];
};
void main()
{
clrscr();
company arr[5];
for(int i=0;i<5;i++)
{
cout<<“enter model name”<<endl;
cin>>arr[i].modelname;
cout<<“enter model number”
cin>>arr[i].modelno;
cout<<“enter the cost of car”;
cin>>arr[i].cost;
cout<<“enter the color of car”;
cin>>arr[i].color;
}
cout<<endl;
for(int j=0;j<5;j++)
{
cout<<“model name: “<<arr[j].modelname<<endl;
cout<<“model number “<<arr[j].modelno<<endl;
cout<<“car cost”<<arr[j].cost<<endl;
cout<<“color of car is :”<<arr[j].color<<endl;
}
getch();
}
Also read here
https://eevibes.com/computing/object-oriented-programming/how-to-implement-stacks-in-c/
How to implement stacks in c++?