Types of inheritance
How many types of inheritance are here in C++? Lets learn about each in detail. As we know that when we use the word “shapes” in geometry then it means that shape can be a rectangle, a square, a triangle or many more. So here I am gonna make a base class as “shape” and then deriving three classes: a circle, a rectangle and a triangle. The example is given below:
class shape
{
protected:
int xCo, yCo;
color fillcolor;
fstyle fillstyle;
public:
shape() : xCo(0), yCo(0), fillcolor(cWHITE),
fillstyle(SOLID_FILL)
{ }
shape(int x, int y, color fc, fstyle fs) :
xCo(x), yCo(y), fillcolor(fc), fillstyle(fs)
{ }
void draw() const
{
set_color(fillcolor);
set_fill_style(fillstyle);
}
};
class circle : public shape
{
private:
int radius;
public:
circle() : shape()
{ }
circle(int x, int y, int r, color fc, fstyle fs)
: shape(x, y, fc, fs), radius(r)
{ }
void draw() const
{
shape::draw();
draw_circle(xCo, yCo, radius);
}
};
////////////////////////////////////////////////////////////////
class rect : public shape
{
private:
int width, height;
public:
rect() : shape(), height(0), width(0)
{ } //6-arg ctor
rect(int x, int y, int h, int w, color fc, fstyle fs) :
shape(x, y, fc, fs), height(h), width(w)
{ }
void draw() const
{
shape::draw();
draw_rectangle(xCo, yCo, xCo+width, yCo+height);
set_color(cWHITE);
draw_line(xCo, yCo, xCo+width, yCo+height);
}
};
class tria : public shape
{
private:
int height;
public:
tria() : shape(), height(0)
{ }
tria(int x, int y, int h, color fc, fstyle fs) :
shape(x, y, fc, fs), height(h)
{ }
void draw() const
{
shape::draw();
draw_pyramid(xCo, yCo, height);
}
};
void main()
{
init_graphics();
circle cir(40, 12, 5, cBLUE, X_FILL);
rect rec(12, 7, 10, 15, cRED, SOLID_FILL);
tria tri(60, 7, 11, cGREEN, MEDIUM_FILL);
cir.draw();
rec.draw();
tri.draw();
set_cursor_pos(1, 25);
getch();
}
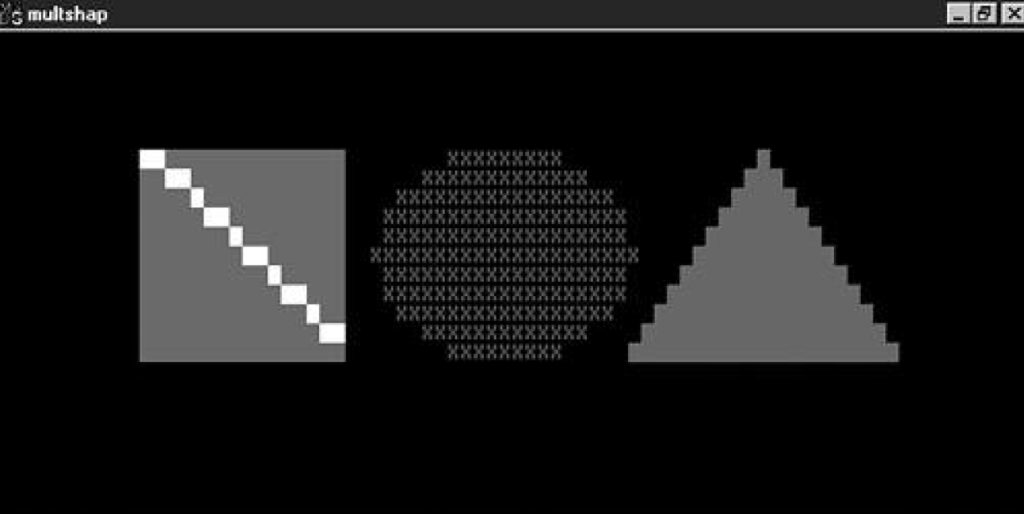
In this above program the shape class has common attributes that are: filling pattern, location, color. The derived classes have then different attributes. For example a triangle has vertex, a rectangle has height and width, while a circle has its radius. Here base class is an example of “abstract class”.
Also read here:
https://eevibes.com/what-is-the-inheritance-in-c/